Angular | Working with Angular Examples
Inhaltsverzeichnis
General
On the Angular Website, there are a lot of examples.
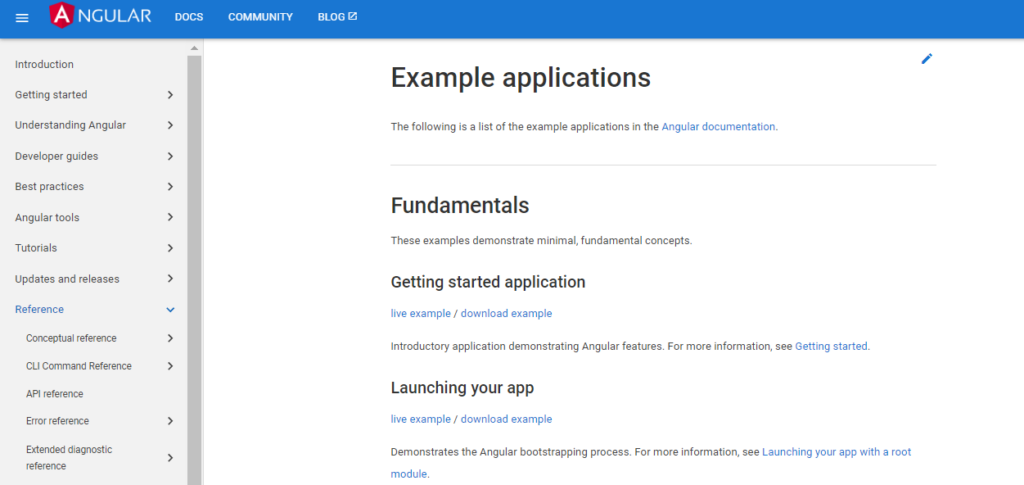
You could download each of them and look around. In this post, i will show you to do this at once for all examples.
So, we will
- get list of all examples
- download zip files of examples
- extract examples
Preparation
First, we define some variables for the Chrom Browser and the resulting output files.
# Mac OS CHROME=/Applications/Chromium.app/Contents/MacOS/Chromium # Windows WSL CHROME='/mnt/c/Program Files/Google/Chrome/Application/chrome.exe' LISTOFZIPS=listofzips
Download List of examples
Next, we will download the list of examples from https://angular.io/guide/example-apps-list.
This is not a static html file. The list will be created dynamically when viewing the page. So, only download the page with
or wget
curl
does
not help, because we need to run the JavaScript code in the browser to create the list.
Therefore, we will use Chrome in headless mode: Load the page and dump the contents of the page (dom) into a file
do_get_list() { "$CHROME" --headless --dump-dom https://angular.io/guide/example-apps-list | tr '>' '>\n ' >${LISTOFZIPS}.html echo "##. Download List of Examples" echo " #Examples: ($(grep 'href="guide/' listofzips.html | wc -l))" }
Then, we will extract all the links to the zip examples files.
A quick look into the html file shows, that the html code with the links looks like this:
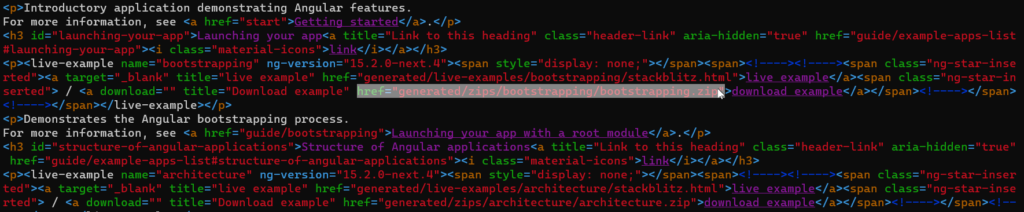
We need the part in the href
of the <a
tag.
- First, we will split the html file, so that every html tag will be on a separate line.
- Then, we will cut out only the parts between apostrophes.
- Then, we search all files containing “.zip” and the end of the line ($)
do_extract() { cat ${LISTOFZIPS}.html |\ tr ' ' '\n' |\ cut -d \" -f2 |\ grep -e '.zip$' > ${LISTOFZIPS} echo "generated/zips/component-overview/component-overview.zip" >> ${LISTOFZIPS} echo "##. Extract zip files ($(wc -l ${LISTOFZIPS}))" echo " #Examples: ($(wc -l ${LISTOFZIPS}))" }
The result will be the list of URL’s for each example.
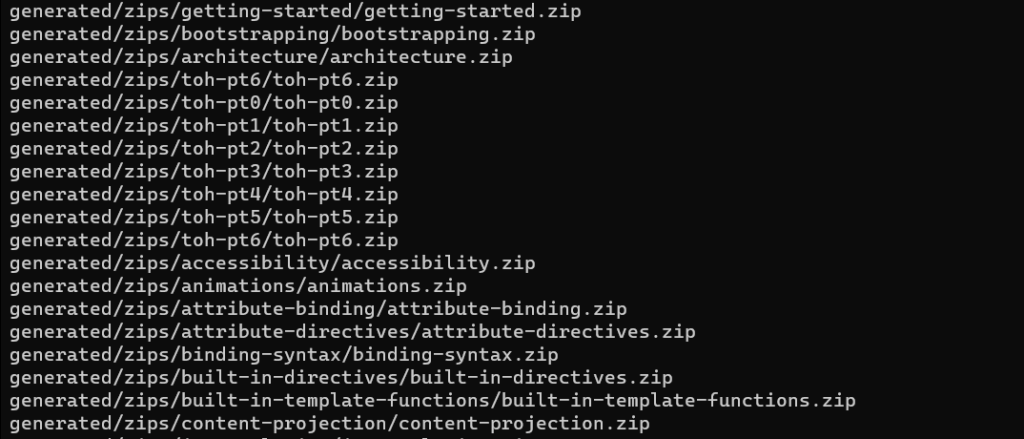
Download Examples
Next, for each of these example $ZIP, we will download the zip from the Angular Website with wget -q https://angular.io/$ZIP
do_download() { echo "##. Download Zips" ( rm -rf zip mkdir -p zip cd zip cat ../$LISTOFZIPS | while read ZIP do echo "Download $ZIP" if [ ! -f $ZIP ]; then wget -q https://angular.io/$ZIP fi done rm *.zip.1 ) }
Extract ZIP Files
do_unzip() { echo "##. Unzip" rm -rf src mkdir -p src find zip -type f -exec basename {} \; | \ while read FILE do FLDR=${FILE/.zip/} echo unzip zip/$FILE -d src/$FLDR unzip zip/$FILE -d src/$FLDR done }
Upgrade all node modules to current version
We will need toe tool npm-check-updates for this.
Install it with
npm install -g npm-check-updates
ncu
updates all packages to the latest version. Some Angular Examples require an earlier version of Typescript, so, after upgrade i changes the Typescript Version to 4.9.5
do_upgrade() { echo "##. Upgrade" ( cd src ls | \ while read FLDR do echo " $FLDR" ( cd $FLDR ncu -u # "typescript": "~4.9.3" sed -i '1,$s/"typescript": ".*"/"typescript": "~4.9.5"/' package.json # pnpm install ) done ) }
Appendix
The code for the complete script is here:
#!/bin/bash # Mac OS CHROME=/Applications/Chromium.app/Contents/MacOS/Chromium # Windows WSL CHROME='/mnt/c/Program Files/Google/Chrome/Application/chrome.exe' LISTOFZIPS=listofzips do_get_list() { "$CHROME" --headless --dump-dom https://angular.io/guide/example-apps-list | tr '>' '>\n ' >${LISTOFZIPS}.html echo "##. Download List of Examples" echo " #Examples: ($(grep 'href="guide/' listofzips.html | wc -l))" } do_extract() { cat ${LISTOFZIPS}.html |\ tr ' ' '\n' |\ cut -d \" -f2 |\ grep -e '.zip$' > ${LISTOFZIPS} echo "generated/zips/component-overview/component-overview.zip" >> ${LISTOFZIPS} echo "##. Extract zip files ($(wc -l ${LISTOFZIPS}))" echo " #Examples: ($(wc -l ${LISTOFZIPS}))" } do_download() { echo "##. Download Zips" ( rm -rf zip mkdir -p zip cd zip cat ../$LISTOFZIPS | while read ZIP do echo "Download $ZIP" if [ ! -f $ZIP ]; then wget -q https://angular.io/$ZIP fi done rm *.zip.1 ) } do_unzip() { echo "##. Unzip" rm -rf src mkdir -p src find zip -type f -exec basename {} \; | \ while read FILE do FLDR=${FILE/.zip/} echo unzip zip/$FILE -d src/$FLDR unzip zip/$FILE -d src/$FLDR done } do_upgrade() { echo "##. Upgrade" ( cd src ls | \ while read FLDR do echo " $FLDR" ( cd $FLDR ncu -u # "typescript": "~4.9.3" sed -i '1,$s/"typescript": ".*"/"typescript": "~4.9.5"/' package.json # pnpm install ) done ) } case "$1" in "get") do_get_list;; "extract") do_extract;; "download") do_download;; "unzip") do_unzip;; "upgrade") do_upgrade;; *) echo "run $0 get|extract|download|unzip";; esac
Leave a Reply